Monday, 23 March 2020
Building Serverless CRUD services in Go with DynamoDB - Part 5
Welcome to Part 5. Last time we've learnt how to delete a single item in a table by primary key. In this post, we'll learn how to secure our APIs.
# Getting started
First, let's add the config under functions in serverless.yml
```
auth:
package:
include:
- ./bin/handlers/authHandler
handler: bin/handlers/authHandler
```
Previously, we've already created ``list``, ``create``, ``update`` and ``delete``. We would like to secure these APIs. To do so, we just need to simply to just add ``authorizer: auth`` to allow us to run an AWS Lambda Function before your targeted AWS Lambda Function.
Take ``list`` as an example:
```
list:
handler: bin/handlers/listHandler
package:
include:
- ./bin/handlers/listHandler
events:
- http:
path: iam
method: get
cors: true
authorizer: auth
```
Then also add it to ``create``, ``update`` and ``delete``.
Before running our business logic, we can perform some Authorisation. It's also useful for micro-service Architectures.
The next step is to create ``authHandler.go`` under ``src/handlers/``. We need a custom authoriser calling an AWS Lambda Function.
About few months ago, I wrote a tutorial to teach how to build a simple authoriser. If you miss it, please check it out via below link and come back later. We'll use the exact code for this tutorial.
{% link https://dev.to/wingkwong/a-simple-amazon-api-gateway-lambda-authoriser-in-go-4cgd %}
In this example, our authentication strategy is to use bearer token like JWT to authorise our requests before reaching to our endpoints.
Under your environment in serverless.yml, you should add your JWT_SECRET_KEY.
```
environment:
IAM_TABLE_NAME: ${self:custom.iamTableName}
JWT_SECRET_KEY:
```
Run the below command to deploy our code
```
./scripts/deploy.sh
```
# Testing
Go to Amazon API Gateway Console, Select your API and Click Authorizers.
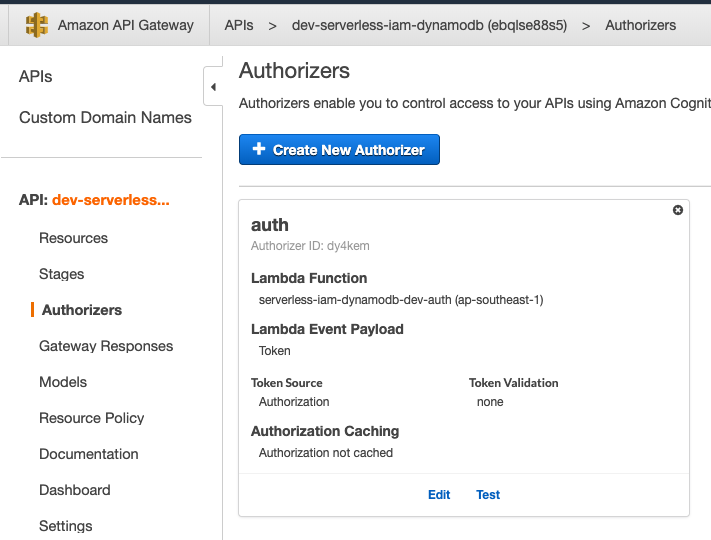
If you test it without the token, you are expected to see the below messages.
```
Response
Response Code: 401
Latency 344
Execution log for request [HIDDEN]
Mon Dec 30 08:56:58 UTC 2019 : Starting authorizer: [HIDDEN]
Mon Dec 30 08:56:58 UTC 2019 : Incoming identity: [HIDDEN]
Mon Dec 30 08:56:58 UTC 2019 : Endpoint request URI: [HIDDEN]
Mon Dec 30 08:56:58 UTC 2019 : Endpoint request headers: [HIDDEN]
Mon Dec 30 08:56:58 UTC 2019 : Endpoint request body after transformations: [HIDDEN]
Mon Dec 30 08:56:58 UTC 2019 : Sending request to [HIDDEN]
Mon Dec 30 08:56:58 UTC 2019 : Unauthorized request: [HIDDEN]
Mon Dec 30 08:56:58 UTC 2019 : Unauthorized
```
With the token, you should see the policy statement authorise our requests.
```json
{
"Version": "2012-10-17",
"Statement": [
{
"Action": [
"execute-api:Invoke"
],
"Effect": "Allow",
"Resource": [
"arn:aws:execute-api:ap-southeast-1:*:a123456789/ESTestInvoke-stage/GET/"
]
}
]
}
```
That's it for part 5. In the next part, we'll create ``loginHandler.go``.
Subscribe to:
Post Comments (Atom)
A Fun Problem - Math
# Problem Statement JATC's math teacher always gives the class some interesting math problems so that they don't get bored. Today t...
-
SHA stands for Secure Hashing Algorithm and 2 is just a version number. SHA-2 revises the construction and the big-length of the signature f...
-
Contest Link: [https://www.e-olymp.com/en/contests/19775](https://www.e-olymp.com/en/contests/19775) Full Solution: [https://github.com/...
No comments:
Post a Comment