Friday 20 March 2020
Building Serverless CRUD services in Go with DynamoDB - Part 4
Welcome back! It's been a while. Here's the part 4. In this post, we will create ``deleteHandler.go``.
# Getting started
First, let's add the config under functions in serverless.yml
```
delete:
handler: bin/handlers/deleteHandler
package:
include:
- ./bin/handlers/deleteHandler
events:
- http:
path: iam/{id}
method: delete
cors: true
```
Create a file deleteHandler.go under src/handlers
Similarly, we have the below structure.
```
package main
import (
"context"
"fmt"
"github.com/aws/aws-lambda-go/events"
"github.com/aws/aws-lambda-go/lambda"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/dynamodb"
"os"
)
var svc *dynamodb.DynamoDB
func init() {
region := os.Getenv("AWS_REGION")
// Initialize a session
if session, err := session.NewSession(&aws.Config{
Region: ®ion,
}); err != nil {
fmt.Println(fmt.Sprintf("Failed to initialize a session to AWS: %s", err.Error()))
} else {
// Create DynamoDB client
svc = dynamodb.New(session)
}
}
func Delete(ctx context.Context, request events.APIGatewayProxyRequest) (events.APIGatewayProxyResponse, error) {
var (
tableName = aws.String(os.Getenv("IAM_TABLE_NAME"))
id = aws.String(request.PathParameters["id"])
)
// TODO: Add delete logic
}
func main() {
lambda.Start(Delete)
}
```
Deleting a record is pretty simple, we just need the record id (primary key) which can be retrieved from the request path parameters ``id``.
```
func (c *DynamoDB) DeleteItem(input *DeleteItemInput) (*DeleteItemOutput, error)
```
In order to call DeleteItem API operation for Amazon DynamoDB, we need to build ``DeleteItemInput`` first.
```
input := &dynamodb.DeleteItemInput{
Key: map[string]*dynamodb.AttributeValue{
"id": {
S: id,
},
},
TableName: tableName,
}
```
If you define a composite primary key, you must provide values for both the partition key and the sort key. In this case, we just need to provide the first one. We also need to tell which table your records are located in.
call ``DeleteItem`` to delete a single item in a table by primary key
```
_, err := svc.DeleteItem(input)
if err != nil {
fmt.Println("Got error calling DeleteItem:")
fmt.Println(err.Error())
// Status Internal Server Error
return events.APIGatewayProxyResponse{
Body: err.Error(),
StatusCode: 500,
}, nil
}
// Status No Content
return events.APIGatewayProxyResponse{
StatusCode: 204,
}, nil
```
Run the below command to deploy our code
```
./scripts/deploy.sh
```
# Testing
If you go to AWS Lambda Console, you will see there is a function called ``serverless-iam-dynamodb-dev-delete``
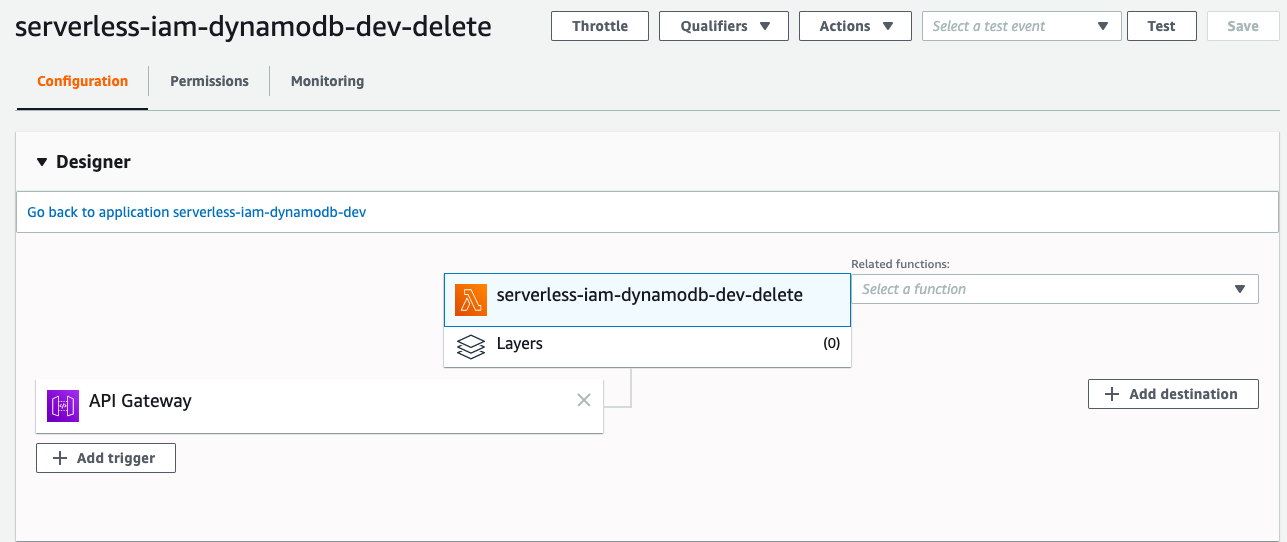
You can test your code either in Lambda or API Gateway.
Upon the success deletion, you should see that the status code returns 204.
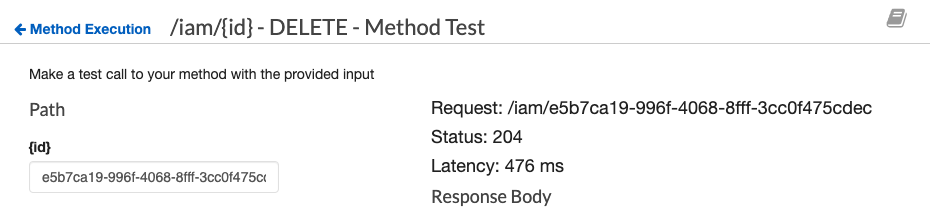
That's it for part 4. In the next post, we'll create ``authHandler.go`` to secure our APIs.
Subscribe to:
Post Comments (Atom)
A Fun Problem - Math
# Problem Statement JATC's math teacher always gives the class some interesting math problems so that they don't get bored. Today t...
-
SHA stands for Secure Hashing Algorithm and 2 is just a version number. SHA-2 revises the construction and the big-length of the signature f...
-
Contest Link: [https://www.e-olymp.com/en/contests/19775](https://www.e-olymp.com/en/contests/19775) Full Solution: [https://github.com/...
No comments:
Post a Comment