Sunday, 29 December 2019
Building a Quiz with React using react-quiz-component
## Initial Setup
The first step is to install the library. Installing react-quiz-component is pretty simple. You just need to install it via npm:
```
npm i react-quiz-component
```
After that, you have to import Quiz class to your project:
```
import Quiz from 'react-quiz-component';
```
## Defining your quiz source
The next step is to define a quiz source in a JSON format. If you are not familiar with JSON, I have created another project called react-quiz-form to generate the JSON string with some validations.

Once you have submitted, you will get the JSON string like this:
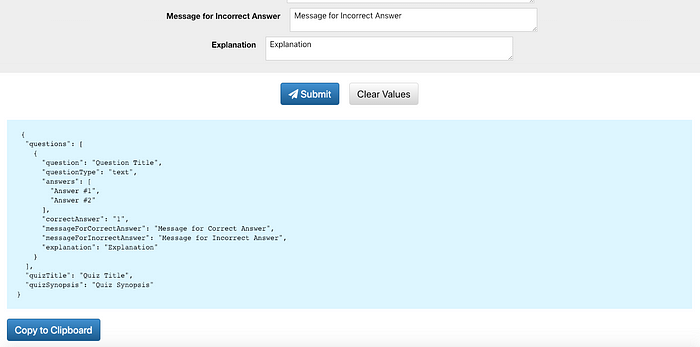
A quiz object may contains three attributes: ``quizTitle`` , ``quizSynopsis`` and ``questions``.
You do not need to include quizSynopsis but it is recommended to include it to let your users to know more about your quiz.
For questions, it may include a single or multiple objects defining the ``question``, ``questionType``, ``answers``, ``correctAnswer``, ``messageForCorrectAnswer``, ``messageForIncorrectAnswer``, and ``explanation``.
### question
The question you want to ask your users.
```
"question": "How can you access the state of a component from inside of a member function?"
```
### questionType
The type of your question. Supported types include text and photo.
```
"questionType": "text"
```
or
```
"questionType": "photo"
```
### answers
The possible answers for users to select.
For ``questionType`` is ``text`` :
```
"answers": [
"this.getState()",
"this.prototype.stateValue",
"this.state",
"this.values"
]
```
For ``questionType`` is ``photo`` :
```
"answers": [
"https://dummyimage.com/600x400/000/fff&text=A",
"https://dummyimage.com/600x400/000/fff&text=B",
"https://dummyimage.com/600x400/000/fff&text=C",
"https://dummyimage.com/600x400/000/fff&text=D"
]
```
### correctAnswer
The index of the correct answer (starting with 1). Current multiple answers are not supported.
```
"correctAnswer": "3"
```
### messageForCorrectAnswer
The message shown when the user answered correctly.
```
"messageForCorrectAnswer": "Correct answer. Good job."
```
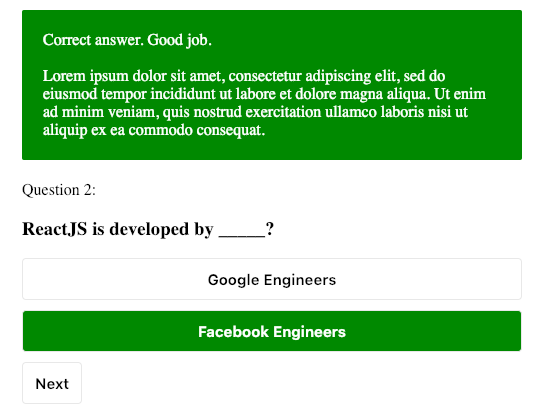
### messageForIncorrectAnswer
The message shown when the user answered incorrectly.
```
"messageForIncorrectAnswer": "Incorrect answer. Please try again."
```
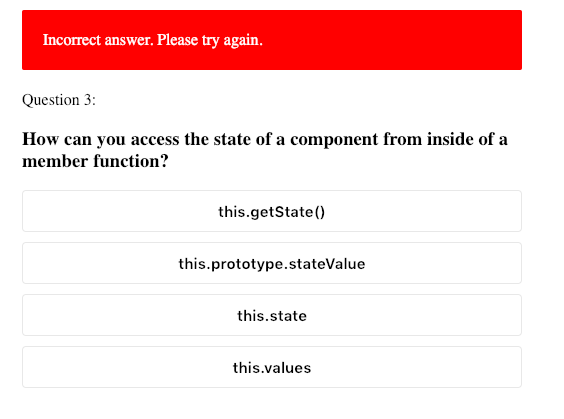
### explanation
The explanation for this question.
```
"explanation": "Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat."
```
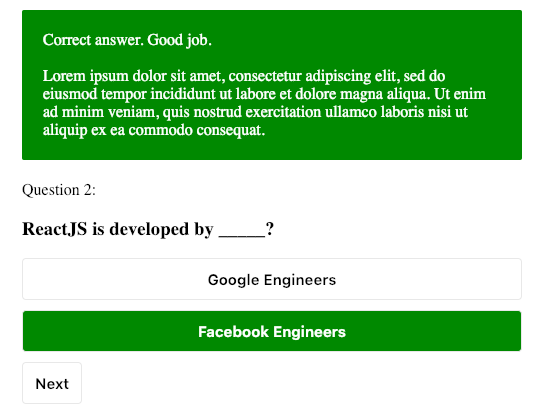
You may create a file called quiz.js. A full example is shown as below:
```
export const quiz = {
"quizTitle": "React Quiz Component Demo",
"quizSynopsis": "Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Aenean commodo ligula eget dolor. Aenean massa. Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus. Donec quam felis, ultricies nec, pellentesque eu, pretium quis, sem. Nulla consequat massa quis enim",
"questions": [
{
"question": "How can you access the state of a component from inside of a member function?",
"questionType": "text",
"answers": [
"this.getState()",
"this.prototype.stateValue",
"this.state",
"this.values"
],
"correctAnswer": "3",
"messageForCorrectAnswer": "Correct answer. Good job.",
"messageForIncorrectAnswer": "Incorrect answer. Please try again.",
"explanation": "Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat."
},
{
"question": "ReactJS is developed by _____?",
"questionType": "text",
"answers": [
"Google Engineers",
"Facebook Engineers"
],
"correctAnswer": "2",
"messageForCorrectAnswer": "Correct answer. Good job.",
"messageForIncorrectAnswer": "Incorrect answer. Please try again.",
"explanation": "Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat."
},
{
"question": "ReactJS is an MVC based framework?",
"questionType": "text",
"answers": [
"True",
"False"
],
"correctAnswer": "2",
"messageForCorrectAnswer": "Correct answer. Good job.",
"messageForIncorrectAnswer": "Incorrect answer. Please try again.",
"explanation": "Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat."
},
{
"question": "Which of the following concepts is/are key to ReactJS?",
"questionType": "text",
"answers": [
"Component-oriented design",
"Event delegation model",
"Both of the above",
],
"correctAnswer": "3",
"messageForCorrectAnswer": "Correct answer. Good job.",
"messageForIncorrectAnswer": "Incorrect answer. Please try again.",
"explanation": "Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat."
},
{
"question": "Lorem ipsum dolor sit amet, consectetur adipiscing elit,",
"questionType": "photo",
"answers": [
"https://dummyimage.com/600x400/000/fff&text=A",
"https://dummyimage.com/600x400/000/fff&text=B",
"https://dummyimage.com/600x400/000/fff&text=C",
"https://dummyimage.com/600x400/000/fff&text=D"
],
"correctAnswer": "1",
"messageForCorrectAnswer": "Correct answer. Good job.",
"messageForIncorrectAnswer": "Incorrect answer. Please try again.",
"explanation": "Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat."
}
]
}
```
## Passing your quiz object to Quiz container
Once you have defined your quiz object, the next step is to pass it as a prop to Quiz container.
```
import { quiz } from './quiz';
...
```
If you want your questions shuffled every time the component renders, you may simply pass true to the prop shuffle.
```
```
That’s it. With these steps, you have integrated ``react-quiz-component`` to your project.
## Quiz Result
``react-quiz-component`` also provides an overall result at the end of the quiz.

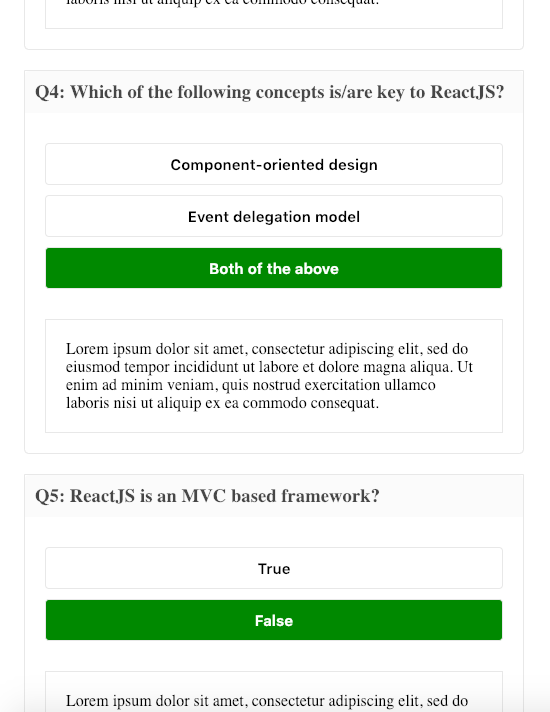
With the dropdown option, you can filter all questions and those that you answered correctly or incorrectly.
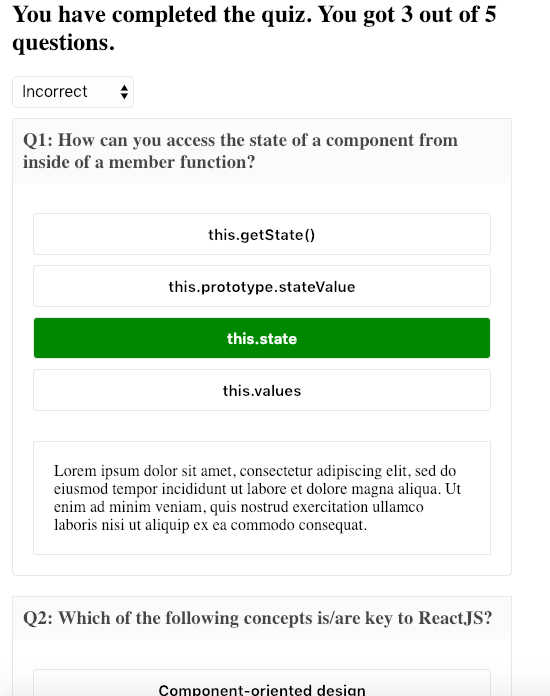
## Demonstration
The demonstration is available at [here](https://wingkwong.github.io/react-quiz-component/)
## Conclusion
react-quiz-component is an open source project. You can easily integrate it with your project. If you found any issues or have some new requested features, please feel free to let me know by filing an issue on the repo shown [here](https://github.com/wingkwong/react-quiz-component).
## Update:
react-quiz-component has introduced more features. For the changelog, please check it [here](https://github.com/wingkwong/react-quiz-component/blob/master/CHANGELOG.md).
Subscribe to:
Post Comments (Atom)
A Fun Problem - Math
# Problem Statement JATC's math teacher always gives the class some interesting math problems so that they don't get bored. Today t...
-
SHA stands for Secure Hashing Algorithm and 2 is just a version number. SHA-2 revises the construction and the big-length of the signature f...
-
Contest Link: [https://www.e-olymp.com/en/contests/19775](https://www.e-olymp.com/en/contests/19775) Full Solution: [https://github.com/...
No comments:
Post a Comment